Comparing two strings lexicographically in C++ is a common task in programming, especially when dealing with sorting algorithms, data structures, and text processing. COMPARE.EDU.VN provides a detailed comparison of different methods to accomplish this, enabling you to choose the most efficient and suitable approach for your specific needs. Understanding string comparison, string ordering, and lexical comparison is crucial for effective programming.
1. Understanding Lexicographical String Comparison
Lexicographical comparison, also known as dictionary order, involves comparing strings based on the ASCII values of their characters. This method is fundamental for sorting strings and implementing search algorithms.
1.1. What is Lexicographical Order?
Lexicographical order arranges strings as they would appear in a dictionary. It compares characters from left to right until a difference is found. The string with the smaller character (based on ASCII value) comes first. If one string is a prefix of another, the shorter string is considered smaller.
For example:
- “apple” comes before “banana” because ‘a’ comes before ‘b’.
- “apple” comes before “apple pie” because “apple” is a prefix of “apple pie.”
- “cat” comes before “caterpillar”
1.2. Importance of Lexicographical Comparison
Lexicographical comparison is important because:
- Sorting: It’s used in sorting algorithms to arrange strings alphabetically.
- Searching: It helps in implementing efficient search algorithms in data structures like trees and dictionaries.
- Data Validation: It’s used to validate input strings against predefined rules.
- Text Processing: It’s used in various text processing tasks, such as text indexing and searching.
2. Methods to Compare Strings Lexicographically in C++
C++ offers several methods for comparing strings lexicographically, each with its own advantages and use cases. These include using the compare()
function, strcmp()
function, and comparison operators.
2.1. Using the compare()
Function in C++ Strings
The C++ string class provides a compare()
function that offers a versatile way to compare two strings. This function returns an integer value indicating the relationship between the strings.
2.1.1. Syntax of compare()
The syntax for the compare()
function is:
int string1.compare(string2);
Here, string1
and string2
are the strings you want to compare. The function returns:
- 0 if
string1
is equal tostring2
. - A negative value if
string1
is less thanstring2
. - A positive value if
string1
is greater thanstring2
.
2.1.2. Example Using compare()
#include <iostream>
#include <string>
using namespace std;
string solve(string s, string t) {
int ret = s.compare(t);
if (ret == 0) {
return s + " and " + t + " are the same";
} else if (ret > 0) {
return s + " is larger than " + t;
} else {
return s + " is smaller than " + t;
}
}
int main() {
string s = "apple";
string t = "appeal";
cout << "The result of comparison: " << solve(s, t) << endl;
s = "popular";
t = "popular";
cout << "The result of comparison: " << solve(s, t) << endl;
s = "Hello";
t = "hello";
cout << "The result of comparison: " << solve(s, t) << endl;
return 0;
}
This example demonstrates how to use the compare()
function to compare strings and determine their lexicographical order.
2.1.3. Advantages of Using compare()
- Clarity: The function explicitly indicates the comparison operation.
- Flexibility: It offers overloaded versions for comparing substrings or portions of strings.
- Standard Library: It’s part of the C++ standard library, ensuring portability and compatibility.
2.2. Using strcmp()
Function in C-like Strings
In C++, you can also use the strcmp()
function from the C standard library to compare C-style strings (character arrays). This function is useful when working with legacy code or when you need to interface with C libraries.
2.2.1. Syntax of strcmp()
The syntax for the strcmp()
function is:
int strcmp(const char* str1, const char* str2);
Here, str1
and str2
are pointers to C-style strings. The function returns:
- 0 if
str1
is equal tostr2
. - A negative value if
str1
is less thanstr2
. - A positive value if
str1
is greater thanstr2
.
2.2.2. Example Using strcmp()
#include <iostream>
#include <cstring>
#include <string>
using namespace std;
string solve(const char* s, const char* t) {
int ret = strcmp(s, t);
if (ret == 0) {
return string(s) + " and " + string(t) + " are the same";
} else if (ret > 0) {
return string(s) + " is larger than " + string(t);
} else {
return string(s) + " is smaller than " + string(t);
}
}
int main() {
string s = "apple";
string t = "appeal";
cout << "The result of comparison: " << solve(s.c_str(), t.c_str()) << endl;
s = "popular";
t = "popular";
cout << "The result of comparison: " << solve(s.c_str(), t.c_str()) << endl;
s = "Hello";
t = "hello";
cout << "The result of comparison: " << solve(s.c_str(), t.c_str()) << endl;
return 0;
}
This example demonstrates how to use the strcmp()
function to compare C-style strings. Note that you need to convert C++ strings to C-style strings using the c_str()
method.
2.2.3. Considerations When Using strcmp()
- Null Termination:
strcmp()
relies on null-terminated strings. Ensure your strings are properly terminated. - Safety: Be cautious of buffer overflows when using
strcmp()
with user-supplied input. - C-style Strings: This function is designed for C-style strings, not C++ string objects directly.
2.3. Using Comparison Operators
C++ allows you to use comparison operators directly on string objects. This method is often the most intuitive and concise for comparing strings.
2.3.1. Available Comparison Operators
The following comparison operators can be used with C++ strings:
==
(equal to)!=
(not equal to)>
(greater than)<
(less than)>=
(greater than or equal to)<=
(less than or equal to)
2.3.2. Example Using Comparison Operators
#include <iostream>
#include <string>
using namespace std;
string solve(string s, string t) {
if (s == t) {
return s + " and " + t + " are the same";
} else if (s > t) {
return s + " is larger than " + t;
} else {
return s + " is smaller than " + t;
}
}
int main() {
string s = "apple";
string t = "appeal";
cout << "The result of comparison: " << solve(s, t) << endl;
s = "popular";
t = "popular";
cout << "The result of comparison: " << solve(s, t) << endl;
s = "Hello";
t = "hello";
cout << "The result of comparison: " << solve(s, t) << endl;
return 0;
}
This example demonstrates how to use comparison operators to compare strings directly. The code is more readable and easier to understand.
2.3.3. Advantages of Using Comparison Operators
- Readability: The code is more concise and easier to read.
- Intuitive: The operators directly reflect the comparison being performed.
- Efficiency: In many cases, the compiler can optimize comparison operators for better performance.
3. Detailed Comparison of Methods
To help you choose the best method for comparing strings lexicographically in C++, here’s a detailed comparison of the three approaches:
Feature | compare() Function |
strcmp() Function |
Comparison Operators |
---|---|---|---|
Data Type | C++ Strings | C-style Strings | C++ Strings |
Return Value | Integer (0, < 0, > 0) | Integer (0, < 0, > 0) | Boolean (true/false) |
Readability | Good | Moderate | Excellent |
Flexibility | High | Moderate | Basic |
Standard Compliance | C++ | C | C++ |
Safety | Safe | Potentially unsafe | Safe |
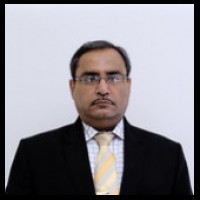
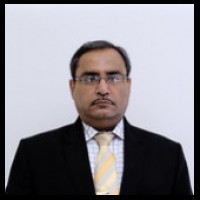
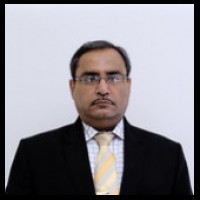
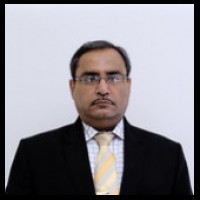
4. Best Practices for Lexicographical String Comparison
When comparing strings lexicographically in C++, consider the following best practices:
- Choose the Right Method: Select the method that best fits your needs based on the type of strings you are working with and the level of control you require.
- Handle Case Sensitivity: Be aware of case sensitivity. If you need to perform a case-insensitive comparison, convert the strings to lowercase or uppercase before comparing.
- Consider Performance: For large-scale comparisons, benchmark different methods to identify the most efficient one.
- Use Standard Library Functions: Prefer standard library functions like
compare()
and comparison operators for better portability and safety. - Validate Inputs: When using
strcmp()
, ensure that the C-style strings are properly null-terminated to prevent unexpected behavior. - Error Handling: Implement appropriate error handling to deal with potential issues, such as invalid input or unexpected string formats.
- Code Clarity: Write code that is easy to read and understand, using meaningful variable names and comments to explain the logic.
5. Case Sensitivity in String Comparison
By default, string comparisons in C++ are case-sensitive. This means that “apple” is different from “Apple”. If you need to perform case-insensitive comparisons, you must convert the strings to the same case before comparing them.
5.1. Converting Strings to Lowercase or Uppercase
You can use the std::transform
function along with std::tolower
or std::toupper
to convert strings to lowercase or uppercase.
5.1.1. Example of Case-Insensitive Comparison
#include <iostream>
#include <string>
#include <algorithm>
#include <cctype>
using namespace std;
string toLower(string s) {
transform(s.begin(), s.end(), s.begin(), ::tolower);
return s;
}
string solveCaseInsensitive(string s, string t) {
s = toLower(s);
t = toLower(t);
if (s == t) {
return s + " and " + t + " are the same";
} else if (s > t) {
return s + " is larger than " + t;
} else {
return s + " is smaller than " + t;
}
}
int main() {
string s = "Apple";
string t = "apple";
cout << "Case-insensitive comparison: " << solveCaseInsensitive(s, t) << endl;
return 0;
}
This example demonstrates how to perform a case-insensitive string comparison by converting both strings to lowercase before comparing them.
5.2. Using Custom Comparison Functions
You can also create custom comparison functions that perform case-insensitive comparisons. These functions can be used with sorting algorithms or other string manipulation tasks.
5.2.1. Example of a Custom Case-Insensitive Comparison Function
#include <iostream>
#include <string>
#include <algorithm>
#include <cctype>
using namespace std;
bool caseInsensitiveCompare(const string& a, const string& b) {
string aLower = a;
string bLower = b;
transform(aLower.begin(), aLower.end(), aLower.begin(), ::tolower);
transform(bLower.begin(), bLower.end(), bLower.begin(), ::tolower);
return aLower < bLower;
}
int main() {
string s1 = "Apple";
string s2 = "banana";
string s3 = "apple";
cout << "Apple < banana (case-insensitive): " << caseInsensitiveCompare(s1, s2) << endl;
cout << "Apple < apple (case-insensitive): " << caseInsensitiveCompare(s1, s3) << endl;
return 0;
}
This example demonstrates how to create a custom comparison function that performs a case-insensitive comparison of two strings.
6. Performance Considerations
The performance of string comparison can be critical in applications that process large amounts of text or perform frequent comparisons. Understanding the performance characteristics of different methods can help you optimize your code.
6.1. Benchmarking String Comparison Methods
Benchmarking involves measuring the execution time of different string comparison methods to determine their performance. You can use the C++ <chrono>
library to measure time intervals accurately.
6.1.1. Example of Benchmarking String Comparison
#include <iostream>
#include <string>
#include <chrono>
#include <cstring>
using namespace std;
using namespace std::chrono;
int main() {
string s1 = "This is a long string for testing performance.";
string s2 = "This is another long string for testing performance.";
// Benchmarking compare()
auto start = high_resolution_clock::now();
for (int i = 0; i < 100000; ++i) {
s1.compare(s2);
}
auto stop = high_resolution_clock::now();
auto duration = duration_cast<microseconds>(stop - start);
cout << "compare() duration: " << duration.count() << " microseconds" << endl;
// Benchmarking strcmp()
start = high_resolution_clock::now();
for (int i = 0; i < 100000; ++i) {
strcmp(s1.c_str(), s2.c_str());
}
stop = high_resolution_clock::now();
duration = duration_cast<microseconds>(stop - start);
cout << "strcmp() duration: " << duration.count() << " microseconds" << endl;
// Benchmarking comparison operators
start = high_resolution_clock::now();
for (int i = 0; i < 100000; ++i) {
s1 == s2;
}
stop = high_resolution_clock::now();
duration = duration_cast<microseconds>(stop - start);
cout << "Comparison operators duration: " << duration.count() << " microseconds" << endl;
return 0;
}
This example demonstrates how to benchmark different string comparison methods using the <chrono>
library. The results can help you identify the most efficient method for your specific use case.
6.2. Factors Affecting Performance
Several factors can affect the performance of string comparison, including:
- String Length: Longer strings take more time to compare.
- String Content: Strings with significant differences early in the comparison process can be compared more quickly.
- Hardware: The speed of the processor and memory can affect performance.
- Compiler Optimization: Compiler optimizations can improve the performance of string comparison.
7. Applications of Lexicographical String Comparison
Lexicographical string comparison is used in a wide range of applications, including:
- Sorting Algorithms: Sorting strings alphabetically.
- Data Structures: Implementing search trees and dictionaries.
- Text Editors: Implementing features like auto-completion and spell-checking.
- Databases: Indexing and searching text fields.
- Command Line Interfaces: Parsing and validating user input.
7.1. Sorting Algorithms
Lexicographical comparison is fundamental to sorting algorithms that arrange strings in alphabetical order. Algorithms like quicksort, mergesort, and heapsort can be used with custom comparison functions to sort strings lexicographically.
7.1.1. Example of Sorting Strings Lexicographically
#include <iostream>
#include <string>
#include <vector>
#include <algorithm>
using namespace std;
int main() {
vector<string> words = {"banana", "apple", "cherry", "date"};
sort(words.begin(), words.end());
cout << "Sorted words: ";
for (const string& word : words) {
cout << word << " ";
}
cout << endl;
return 0;
}
This example demonstrates how to use the std::sort
function to sort a vector of strings lexicographically.
7.2. Data Structures
Lexicographical comparison is used in data structures like search trees and dictionaries to organize and search for strings efficiently.
7.2.1. Example of Using Lexicographical Comparison in a Binary Search Tree
#include <iostream>
#include <string>
using namespace std;
struct Node {
string data;
Node* left;
Node* right;
Node(string data) : data(data), left(nullptr), right(nullptr) {}
};
Node* insert(Node* root, string data) {
if (root == nullptr) {
return new Node(data);
}
if (data < root->data) {
root->left = insert(root->left, data);
} else {
root->right = insert(root->right, data);
}
return root;
}
void inorderTraversal(Node* root) {
if (root != nullptr) {
inorderTraversal(root->left);
cout << root->data << " ";
inorderTraversal(root->right);
}
}
int main() {
Node* root = nullptr;
root = insert(root, "banana");
root = insert(root, "apple");
root = insert(root, "cherry");
root = insert(root, "date");
cout << "Inorder traversal: ";
inorderTraversal(root);
cout << endl;
return 0;
}
This example demonstrates how to use lexicographical comparison to insert strings into a binary search tree, ensuring that the strings are organized in alphabetical order.
8. Advanced Techniques
For more advanced string comparison scenarios, consider the following techniques:
8.1. Using Locale-Specific Comparisons
C++ locales allow you to perform string comparisons based on specific regional settings. This can be useful when dealing with strings in different languages or character sets.
8.1.1. Example of Using Locale-Specific Comparison
#include <iostream>
#include <string>
#include <locale>
#include <algorithm>
using namespace std;
int main() {
locale german("de_DE.UTF-8");
string s1 = "äpfel";
string s2 = "apfel";
cout << "Default comparison: " << (s1 < s2) << endl;
// Using locale-specific comparison
cout << "Locale-specific comparison: " << (use_facet<collate<char>>(german).compare(s1.c_str(), s1.c_str() + s1.length(),
s2.c_str(), s2.c_str() + s2.length()) < 0) << endl;
return 0;
}
This example demonstrates how to use locale-specific comparisons to handle strings with characters specific to the German language.
8.2. Using Regular Expressions
Regular expressions provide a powerful way to perform complex string matching and comparison. You can use regular expressions to validate string formats, search for patterns, and perform advanced text processing tasks.
8.2.1. Example of Using Regular Expressions for String Comparison
#include <iostream>
#include <string>
#include <regex>
using namespace std;
int main() {
string s = "The quick brown fox";
regex pattern("quick.*fox");
cout << "String matches pattern: " << regex_search(s, pattern) << endl;
return 0;
}
This example demonstrates how to use regular expressions to search for a specific pattern in a string.
9. Common Pitfalls and How to Avoid Them
When comparing strings lexicographically in C++, be aware of the following common pitfalls and how to avoid them:
- Case Sensitivity: Always consider case sensitivity and convert strings to the same case if necessary.
- Null Termination: Ensure that C-style strings are properly null-terminated to prevent buffer overflows.
- Locale Issues: Be aware of locale-specific character sets and use appropriate comparison methods when dealing with internationalized strings.
- Performance Bottlenecks: Monitor the performance of string comparison and optimize your code if necessary.
10. FAQs About Lexicographical String Comparison in C++
Here are some frequently asked questions about lexicographical string comparison in C++:
-
What is lexicographical order?
Lexicographical order is the order in which strings would appear in a dictionary, based on the ASCII values of their characters. -
How do I compare strings in C++?
You can use thecompare()
function,strcmp()
function, or comparison operators to compare strings in C++. -
Is string comparison case-sensitive in C++?
Yes, string comparison is case-sensitive by default. You can convert strings to lowercase or uppercase to perform case-insensitive comparisons. -
What is the difference between
compare()
andstrcmp()
?
Thecompare()
function is used for C++ strings, whilestrcmp()
is used for C-style strings (character arrays). -
How do I perform a case-insensitive string comparison?
Convert both strings to lowercase or uppercase before comparing them. -
Which method is the most efficient for string comparison?
The efficiency depends on the specific use case. Comparison operators are often the most efficient for simple comparisons. -
What are the common pitfalls in string comparison?
Common pitfalls include case sensitivity, null termination issues, and locale issues. -
Can I use regular expressions for string comparison?
Yes, regular expressions can be used for complex string matching and comparison. -
How do I sort strings lexicographically?
You can use thestd::sort
function with a custom comparison function to sort strings lexicographically. -
Why is lexicographical string comparison important?
It is important for sorting, searching, data validation, and text processing tasks.
11. Conclusion: Choosing the Right Method for String Comparison
In conclusion, knowing How To Compare Two Strings Lexicographically In C++ is essential for any programmer. Whether you are sorting data, validating input, or processing text, the ability to compare strings effectively is crucial. By understanding the different methods available—compare()
function, strcmp()
function, and comparison operators—you can choose the best approach for your specific needs. Always consider case sensitivity, performance, and the type of strings you are working with to write efficient and reliable code.
At COMPARE.EDU.VN, we understand the challenges of making informed decisions. That’s why we provide comprehensive and objective comparisons of various tools and techniques. If you’re struggling to decide which string comparison method is best for your project, visit COMPARE.EDU.VN. Our detailed analyses and user reviews will help you make the right choice.
For more information, visit our website at compare.edu.vn or contact us at 333 Comparison Plaza, Choice City, CA 90210, United States, or via Whatsapp at +1 (626) 555-9090. Let us help you make the best decisions for your programming needs by comparing string functions, string methods and string utilities today.