Comparing dates in SQL can initially seem complex, especially when you need to ensure the date formats match or when dealing with DateTime values. However, with the right approach, it becomes a straightforward task. This guide, brought to you by COMPARE.EDU.VN, will provide you with a detailed explanation of how to compare dates effectively in SQL, covering various scenarios and techniques using comparison operators and built-in functions. Learn how to perform date comparisons, handle different date formats, and utilize SQL’s date functions to refine your queries and analyses.
1. Understanding SQL Date and DateTime Data Types
SQL uses specific data types to store date and time values. The most common ones are DATE
, DATETIME
, DATETIME2
, SMALLDATETIME
, and TIMESTAMP
. Understanding these data types is crucial for performing accurate date comparisons.
- DATE: Stores only the date part (year, month, and day).
- DATETIME: Stores both date and time components with a higher precision.
- DATETIME2: A newer version of DATETIME with increased range, precision and is timezone aware.
- SMALLDATETIME: Stores date and time with less precision than DATETIME.
- TIMESTAMP: Represents a unique binary value that is automatically updated whenever a row is modified, often used for versioning.
When comparing dates, it’s essential to ensure that you’re comparing compatible data types or converting them appropriately. For example, comparing a DATE
value with a DATETIME
value might require you to extract only the date part from the DATETIME
value.
2. Basic Syntax for Date Comparison in SQL
The fundamental method to compare dates in SQL involves using comparison operators such as equals to (=), not equals to (!= or <>), less than (<), greater than (>), less than or equals to (<=), and greater than or equals to (>=).
The general syntax for comparing dates is as follows:
SELECT column_name
FROM table_name
WHERE date_column comparison_operator 'YYYY-MM-DD';
Here’s a breakdown:
column_name
: The column you want to retrieve.table_name
: The table you’re querying.date_column
: The column containing the dates you want to compare.comparison_operator
: Any of the comparison operators mentioned above.'YYYY-MM-DD'
: The date value you’re comparing against. SQL typically uses theYYYY-MM-DD
format by default.
Example:
Suppose you have a table named Orders
with a column OrderDate
of type DATE
. To find all orders placed after January 1, 2023, you would use the following query:
SELECT OrderID, OrderDate
FROM Orders
WHERE OrderDate > '2023-01-01';
This query selects the OrderID
and OrderDate
from the Orders
table where the OrderDate
is greater than January 1, 2023.
3. Comparing Dates Using Comparison Operators
3.1. Equals To (=) Operator
The equals to operator (=) is used to find records where the date matches a specific value.
Example:
To find all orders placed on January 15, 2023, you can use the following query:
SELECT OrderID, OrderDate
FROM Orders
WHERE OrderDate = '2023-01-15';
This query will return all orders that have an OrderDate
exactly matching January 15, 2023.
3.2. Not Equals To (!= or <>) Operator
The not equals to operator (!= or <>) is used to find records where the date does not match a specific value.
Example:
To find all orders not placed on January 15, 2023, you can use the following query:
SELECT OrderID, OrderDate
FROM Orders
WHERE OrderDate != '2023-01-15';
Or:
SELECT OrderID, OrderDate
FROM Orders
WHERE OrderDate <> '2023-01-15';
These queries will return all orders that have an OrderDate
different from January 15, 2023.
3.3. Less Than (<) Operator
The less than operator (<) is used to find records where the date is earlier than a specific value.
Example:
To find all orders placed before January 1, 2023, you can use the following query:
SELECT OrderID, OrderDate
FROM Orders
WHERE OrderDate < '2023-01-01';
This query will return all orders that have an OrderDate
before January 1, 2023.
3.4. Greater Than (>) Operator
The greater than operator (>) is used to find records where the date is later than a specific value.
Example:
To find all orders placed after January 1, 2023, you can use the following query:
SELECT OrderID, OrderDate
FROM Orders
WHERE OrderDate > '2023-01-01';
This query will return all orders that have an OrderDate
after January 1, 2023.
3.5. Less Than or Equals To (<=) Operator
The less than or equals to operator (<=) is used to find records where the date is earlier than or equal to a specific value.
Example:
To find all orders placed on or before January 1, 2023, you can use the following query:
SELECT OrderID, OrderDate
FROM Orders
WHERE OrderDate <= '2023-01-01';
This query will return all orders that have an OrderDate
on or before January 1, 2023.
3.6. Greater Than or Equals To (>=) Operator
The greater than or equals to operator (>=) is used to find records where the date is later than or equal to a specific value.
Example:
To find all orders placed on or after January 1, 2023, you can use the following query:
SELECT OrderID, OrderDate
FROM Orders
WHERE OrderDate >= '2023-01-01';
This query will return all orders that have an OrderDate
on or after January 1, 2023.
4. Comparing Dates with Time Components
When dealing with DATETIME
or DATETIME2
data types, you often need to compare both the date and time components. This can be more complex, as you might want to compare only the date part or the time part, or both.
4.1. Comparing Full DateTime Values
To compare the full DateTime
values, use the same comparison operators as with DATE
values.
Example:
Suppose you have an Events
table with a EventDateTime
column. To find all events that occurred after January 1, 2023, at 10:00 AM, you can use the following query:
SELECT EventID, EventDateTime
FROM Events
WHERE EventDateTime > '2023-01-01 10:00:00';
This query will return all events that have an EventDateTime
after January 1, 2023, at 10:00 AM.
4.2. Comparing Only the Date Part
If you only want to compare the date part of a DateTime
value, you can use the CAST
function to convert the DateTime
value to a DATE
value.
Example:
To find all events that occurred on January 1, 2023, regardless of the time, you can use the following query:
SELECT EventID, EventDateTime
FROM Events
WHERE CAST(EventDateTime AS DATE) = '2023-01-01';
This query converts the EventDateTime
column to a DATE
value and compares it with January 1, 2023.
4.3. Comparing Date Ranges
Comparing date ranges is a common requirement. You can use the BETWEEN
operator or a combination of comparison operators to achieve this.
Using the BETWEEN Operator
The BETWEEN
operator is used to select values within a given range.
Example:
To find all orders placed between January 1, 2023, and January 31, 2023, you can use the following query:
SELECT OrderID, OrderDate
FROM Orders
WHERE OrderDate BETWEEN '2023-01-01' AND '2023-01-31';
This query will return all orders that have an OrderDate
between January 1, 2023, and January 31, 2023, inclusive.
Using Comparison Operators
You can also use a combination of greater than or equals to (>=) and less than or equals to (<=) operators to define a date range.
Example:
The above query can also be written as:
SELECT OrderID, OrderDate
FROM Orders
WHERE OrderDate >= '2023-01-01' AND OrderDate <= '2023-01-31';
This query achieves the same result as the BETWEEN
operator example.
5. Using SQL Date Functions for Comparison
SQL provides several built-in date functions that can be used to manipulate and compare dates. Some of the most useful functions include DATEADD
, DATEDIFF
, DATEPART
, and GETDATE
.
5.1. DATEADD Function
The DATEADD
function is used to add a specified interval to a date.
Syntax:
DATEADD(datepart, number, date)
datepart
: The part of the date to which the interval is added (e.g.,year
,month
,day
).number
: The interval to add.date
: The date to which the interval is added.
Example:
To find all orders placed within the last 7 days, you can use the following query:
SELECT OrderID, OrderDate
FROM Orders
WHERE OrderDate >= DATEADD(day, -7, GETDATE());
This query uses GETDATE()
to get the current date and DATEADD
to subtract 7 days from it.
5.2. DATEDIFF Function
The DATEDIFF
function is used to find the difference between two dates.
Syntax:
DATEDIFF(datepart, startdate, enddate)
datepart
: The part of the date to use for the difference (e.g.,year
,month
,day
).startdate
: The start date.enddate
: The end date.
Example:
To find all orders placed within the last month, you can use the following query:
SELECT OrderID, OrderDate
FROM Orders
WHERE DATEDIFF(month, OrderDate, GETDATE()) <= 1;
This query calculates the difference in months between the OrderDate
and the current date.
5.3. DATEPART Function
The DATEPART
function is used to extract a specific part of a date.
Syntax:
DATEPART(datepart, date)
datepart
: The part of the date to extract (e.g.,year
,month
,day
).date
: The date from which to extract the part.
Example:
To find all orders placed in January, regardless of the year, you can use the following query:
SELECT OrderID, OrderDate
FROM Orders
WHERE DATEPART(month, OrderDate) = 1;
This query extracts the month part from the OrderDate
and compares it with 1 (January).
5.4. GETDATE Function
The GETDATE
function returns the current date and time.
Example:
To find all orders placed today, you can use the following query:
SELECT OrderID, OrderDate
FROM Orders
WHERE CAST(OrderDate AS DATE) = CAST(GETDATE() AS DATE);
This query casts both the OrderDate
and the current date to DATE
values to compare only the date part.
6. Handling Different Date Formats
SQL databases can store dates in various formats, and it’s important to handle these formats correctly when comparing dates. The default format is usually YYYY-MM-DD
, but you might encounter other formats such as MM/DD/YYYY
or DD-MM-YYYY
.
6.1. Using CONVERT Function
The CONVERT
function is used to convert a date from one format to another.
Syntax:
CONVERT(data_type, expression, style)
data_type
: The data type to convert to (e.g.,DATE
,DATETIME
).expression
: The value to convert.style
: The format style.
Example:
If your OrderDate
column is stored as a string in the format MM/DD/YYYY
, you can convert it to the DATE
format before comparing:
SELECT OrderID, OrderDate
FROM Orders
WHERE CONVERT(DATE, OrderDate, 101) > '2023-01-01';
In this example, 101
is the style code for the MM/DD/YYYY
format.
6.2. Common Date Formats and Styles
Here are some common date formats and their corresponding style codes for the CONVERT
function:
Style Code | Date Format | Example |
---|---|---|
101 | MM/DD/YYYY | 01/15/2023 |
102 | YYYY.MM.DD | 2023.01.15 |
103 | DD/MM/YYYY | 15/01/2023 |
104 | DD.MM.YYYY | 15.01.2023 |
105 | DD-MM-YYYY | 15-01-2023 |
112 | YYYYMMDD | 20230115 |
120 | YYYY-MM-DD hh:mi:ss | 2023-01-15 10:30:00 |
6.3. Best Practices for Handling Date Formats
- Consistency: Ensure that your date formats are consistent throughout your database.
- Standard Format: Use the standard
YYYY-MM-DD
format whenever possible to avoid ambiguity. - Explicit Conversion: When dealing with different formats, explicitly convert the dates to a common format before comparing.
7. Case Studies and Examples
To further illustrate how to compare dates in SQL, let’s look at some practical case studies and examples.
7.1. Finding Customers Who Placed Their First Order in 2022
Suppose you have a Customers
table with a CustomerID
and a FirstOrderDate
column. You want to find all customers who placed their first order in 2022.
SELECT CustomerID, FirstOrderDate
FROM Customers
WHERE DATEPART(year, FirstOrderDate) = 2022;
This query extracts the year part from the FirstOrderDate
and compares it with 2022.
7.2. Finding Orders Shipped Within 3 Days of Order Date
Suppose you have an Orders
table with an OrderDate
and a ShipDate
column. You want to find all orders that were shipped within 3 days of the order date.
SELECT OrderID, OrderDate, ShipDate
FROM Orders
WHERE DATEDIFF(day, OrderDate, ShipDate) <= 3;
This query calculates the difference in days between the OrderDate
and the ShipDate
and compares it with 3.
7.3. Finding Orders Placed on Weekends
Suppose you want to find all orders placed on weekends (Saturday and Sunday).
SELECT OrderID, OrderDate
FROM Orders
WHERE DATEPART(weekday, OrderDate) IN (7, 1);
This query extracts the weekday part from the OrderDate
. In SQL Server, 7 represents Saturday, and 1 represents Sunday.
8. Advanced Techniques for Date Comparison
8.1. Using Stored Procedures for Date Comparisons
Stored procedures can be used to encapsulate complex date comparison logic and make it reusable.
Example:
Create a stored procedure to find all orders placed within a specified date range:
CREATE PROCEDURE GetOrdersByDateRange
@StartDate DATE,
@EndDate DATE
AS
BEGIN
SELECT OrderID, OrderDate
FROM Orders
WHERE OrderDate BETWEEN @StartDate AND @EndDate;
END;
To execute the stored procedure:
EXEC GetOrdersByDateRange '2023-01-01', '2023-01-31';
8.2. Using Functions for Date Calculations
User-defined functions can be used to perform custom date calculations.
Example:
Create a function to calculate the number of business days between two dates:
CREATE FUNCTION BusinessDaysBetween (@StartDate DATE, @EndDate DATE)
RETURNS INT
AS
BEGIN
DECLARE @BusinessDays INT;
SET @BusinessDays = 0;
WHILE @StartDate <= @EndDate
BEGIN
IF DATEPART(weekday, @StartDate) NOT IN (1, 7)
SET @BusinessDays = @BusinessDays + 1;
SET @StartDate = DATEADD(day, 1, @StartDate);
END;
RETURN @BusinessDays;
END;
To use the function:
SELECT dbo.BusinessDaysBetween('2023-01-01', '2023-01-31');
9. Performance Considerations for Date Comparisons
When working with large datasets, date comparisons can impact query performance. Here are some tips to optimize date comparisons:
- Indexing: Ensure that the date columns you’re comparing are indexed. This can significantly speed up query execution.
- Data Types: Use appropriate data types for date columns. Using
DATE
orDATETIME2
is generally more efficient than using string data types. - Avoid Functions in WHERE Clause: Avoid using functions in the
WHERE
clause, as this can prevent the database from using indexes. Instead, perform the calculation outside the query or use computed columns. - Partitioning: For very large tables, consider partitioning the table based on date ranges. This can improve query performance by allowing the database to scan only the relevant partitions.
10. Common Mistakes and How to Avoid Them
10.1. Incorrect Date Formats
Using incorrect date formats is a common mistake. Always ensure that your date formats match the expected format of the database or use the CONVERT
function to convert them explicitly.
10.2. Comparing Different Data Types
Comparing DATE
and DATETIME
values without proper conversion can lead to incorrect results. Always cast the values to the same data type before comparing.
10.3. Ignoring Time Zones
When dealing with dates across different time zones, it’s important to consider the time zone differences and convert the dates to a common time zone before comparing.
10.4. Not Using Indexes
Not using indexes on date columns can significantly slow down query performance. Make sure to create indexes on the date columns you frequently use in comparisons.
11. FAQs About Comparing Dates in SQL
1. How do I compare dates in SQL Server?
You can compare dates in SQL Server using comparison operators such as =, <, >, <=, >=, and BETWEEN. Ensure that the dates are in a compatible format or use the CONVERT function to convert them.
2. How do I compare only the date part of a DateTime value?
Use the CAST function to convert the DateTime value to a DATE value before comparing.
3. How do I find the difference between two dates in SQL?
Use the DATEDIFF function to find the difference between two dates in terms of years, months, days, etc.
4. How do I add days to a date in SQL?
Use the DATEADD function to add a specified number of days to a date.
5. How do I handle different date formats in SQL?
Use the CONVERT function to convert dates from one format to another.
6. How do I compare dates across different time zones?
Convert the dates to a common time zone before comparing. You can use functions like AT TIME ZONE in SQL Server 2016 and later.
7. Can I use indexes to improve the performance of date comparisons?
Yes, creating indexes on date columns can significantly improve query performance.
8. How do I find all records between two dates in SQL?
Use the BETWEEN operator to find all records within a specified date range.
9. How do I extract the year, month, or day from a date in SQL?
Use the DATEPART function to extract specific parts of a date.
10. What is the default date format in SQL?
The default date format in SQL is usually YYYY-MM-DD.
12. Conclusion: Make Informed Decisions with COMPARE.EDU.VN
Comparing dates in SQL is a fundamental skill for data analysis and management. By understanding the different data types, comparison operators, and built-in functions, you can perform accurate and efficient date comparisons. Always consider performance implications and handle different date formats and time zones appropriately.
For more detailed comparisons and to make informed decisions, visit COMPARE.EDU.VN. Our platform offers comprehensive comparisons across various topics, helping you make the best choices for your needs. Whether you’re comparing products, services, or ideas, COMPARE.EDU.VN is your go-to resource for objective and thorough analysis.
Need more help with comparisons? Contact us at:
- Address: 333 Comparison Plaza, Choice City, CA 90210, United States
- WhatsApp: +1 (626) 555-9090
- Website: COMPARE.EDU.VN
Let COMPARE.EDU.VN be your guide to making the best decisions, every time.
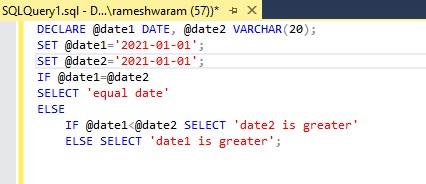{width=462 height=200}
Alt text: SQL query demonstrating date comparison using the equals operator, setting two date variables and comparing if they are equal.
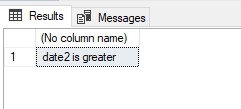{width=241 height=109}
Alt text: Screenshot displaying the result of the SQL query, indicating that ‘date2 is greater’ after comparing two different dates.