Comparing strings lexicographically in C++ is essential for various applications. COMPARE.EDU.VN provides a comprehensive guide to effectively performing string comparisons in C++, offering solutions for determining the order of strings in a dictionary-like manner. Dive in to learn more about string comparison methods, explore efficient code samples, and understand lexicographical ordering, ensuring you make informed decisions.
1. Understanding Lexicographical String Comparison
Lexicographical string comparison, often referred to as dictionary order, involves comparing strings character by character based on their ASCII values. This comparison determines the order in which strings would appear in a dictionary. It’s a fundamental concept in computer science and programming, particularly in tasks such as sorting, searching, and data validation. Understanding how to perform this comparison efficiently in C++ is crucial for developers.
When strings are compared lexicographically, the comparison proceeds from the first character of each string. If the characters are different, the string with the smaller ASCII value is considered lexicographically smaller. If the initial characters are the same, the comparison moves to the next character until a difference is found or one of the strings is exhausted.
1.1. Importance of Lexicographical Order
Lexicographical order plays a vital role in various computing applications. It provides a standardized way to sort and compare strings, ensuring consistency and predictability. Here are some key areas where lexicographical order is essential:
- Sorting Algorithms: Many sorting algorithms rely on lexicographical comparison to arrange strings in a specific order.
- Searching Algorithms: Lexicographical order enables efficient searching within datasets, such as finding a specific word in a dictionary.
- Data Validation: It’s used to validate user inputs, ensuring that data conforms to specific patterns or rules.
- Database Indexing: Databases use lexicographical order to create indexes, improving the speed and efficiency of queries.
- Compiler Design: Lexicographical order is employed in compilers to manage symbols and identifiers.
1.2. Key Principles of Lexicographical Comparison
Understanding the underlying principles of lexicographical comparison is essential for effective implementation. Here are the fundamental rules:
- Character-by-Character Comparison: The comparison starts with the first character of each string.
- ASCII Values: Characters are compared based on their ASCII values. For instance, ‘A’ (ASCII 65) is less than ‘a’ (ASCII 97).
- Prefix Matching: If the initial characters are the same, the comparison moves to the next character. This continues until a difference is found or one string ends.
- Shorter String Priority: If one string is a prefix of the other, the shorter string is considered lexicographically smaller. For example, “apple” is smaller than “applepie”.
- Case Sensitivity: Lexicographical comparison is case-sensitive by default. “apple” is different from “Apple”.
2. Methods for Lexicographical String Comparison in C++
C++ offers several methods to perform lexicographical string comparisons, each with its own advantages and use cases. These methods include using the compare()
function, the strcmp()
function, and comparison operators.
2.1. Using the compare()
Function
The compare()
function is a member function of the C++ string
class, providing a convenient way to compare two strings. It returns an integer value indicating the relationship between the strings:
- 0: If the strings are equal.
- Negative Value: If the first string is lexicographically smaller than the second string.
- Positive Value: If the first string is lexicographically larger than the second string.
2.1.1. Syntax and Usage
The syntax for using the compare()
function is:
string1.compare(string2);
Here’s an example demonstrating how to use the compare()
function:
#include <iostream>
#include <string>
using namespace std;
int main() {
string str1 = "apple";
string str2 = "banana";
string str3 = "apple";
int result1 = str1.compare(str2); // apple vs. banana
int result2 = str1.compare(str3); // apple vs. apple
int result3 = str2.compare(str1); // banana vs. apple
if (result1 < 0) {
cout << str1 << " is smaller than " << str2 << endl;
} else if (result1 > 0) {
cout << str1 << " is larger than " << str2 << endl;
} else {
cout << str1 << " is equal to " << str2 << endl;
}
if (result2 < 0) {
cout << str1 << " is smaller than " << str3 << endl;
} else if (result2 > 0) {
cout << str1 << " is larger than " << str3 << endl;
} else {
cout << str1 << " is equal to " << str3 << endl;
}
if (result3 < 0) {
cout << str2 << " is smaller than " << str1 << endl;
} else if (result3 > 0) {
cout << str2 << " is larger than " << str1 << endl;
} else {
cout << str2 << " is equal to " << str1 << endl;
}
return 0;
}
Output:
apple is smaller than banana
apple is equal to apple
banana is larger than apple
2.1.2. Benefits of Using compare()
- Ease of Use: The
compare()
function is straightforward and easy to use, making it accessible to developers of all levels. - String Class Integration: As a member function of the
string
class, it seamlessly integrates with C++ string objects. - Comprehensive Comparison: It provides a comprehensive comparison, considering all characters in the strings.
2.2. Using the strcmp()
Function
The strcmp()
function is a C-style string comparison function available in C++. It’s part of the <cstring>
library and is used to compare two null-terminated character arrays (C-strings).
2.2.1. Syntax and Usage
The syntax for using the strcmp()
function is:
strcmp(cstr1, cstr2);
Here’s an example demonstrating how to use the strcmp()
function:
#include <iostream>
#include <cstring>
using namespace std;
int main() {
const char* cstr1 = "apple";
const char* cstr2 = "banana";
const char* cstr3 = "apple";
int result1 = strcmp(cstr1, cstr2); // apple vs. banana
int result2 = strcmp(cstr1, cstr3); // apple vs. apple
int result3 = strcmp(cstr2, cstr1); // banana vs. apple
if (result1 < 0) {
cout << cstr1 << " is smaller than " << cstr2 << endl;
} else if (result1 > 0) {
cout << cstr1 << " is larger than " << cstr2 << endl;
} else {
cout << cstr1 << " is equal to " << cstr2 << endl;
}
if (result2 < 0) {
cout << cstr1 << " is smaller than " << cstr3 << endl;
} else if (result2 > 0) {
cout << cstr1 << " is larger than " << cstr3 << endl;
} else {
cout << cstr1 << " is equal to " << cstr3 << endl;
}
if (result3 < 0) {
cout << cstr2 << " is smaller than " << cstr1 << endl;
} else if (result3 > 0) {
cout << cstr2 << " is larger than " << cstr1 << endl;
} else {
cout << cstr2 << " is equal to " << cstr1 << endl;
}
return 0;
}
Output:
apple is smaller than banana
apple is equal to apple
banana is larger than apple
2.2.2. Converting C++ Strings to C-strings
To use strcmp()
with C++ string
objects, you need to convert them to C-strings using the c_str()
method:
#include <iostream>
#include <cstring>
#include <string>
using namespace std;
int main() {
string str1 = "apple";
string str2 = "banana";
int result = strcmp(str1.c_str(), str2.c_str());
if (result < 0) {
cout << str1 << " is smaller than " << str2 << endl;
} else if (result > 0) {
cout << str1 << " is larger than " << str2 << endl;
} else {
cout << str1 << " is equal to " << str2 << endl;
}
return 0;
}
2.2.3. Considerations when using strcmp()
- Null Termination:
strcmp()
relies on null-terminated strings. Ensure that your character arrays are properly null-terminated. - C-style Strings: It works with C-style strings (character arrays) rather than C++
string
objects directly.
2.3. Using Comparison Operators
C++ allows you to use comparison operators (==, !=, >, <, >=, <=) to compare strings lexicographically. These operators are overloaded for the string
class, providing a convenient and intuitive way to compare strings.
2.3.1. Syntax and Usage
Here’s an example demonstrating how to use comparison operators:
#include <iostream>
#include <string>
using namespace std;
int main() {
string str1 = "apple";
string str2 = "banana";
string str3 = "apple";
if (str1 == str2) {
cout << str1 << " is equal to " << str2 << endl;
} else if (str1 < str2) {
cout << str1 << " is smaller than " << str2 << endl;
} else {
cout << str1 << " is larger than " << str2 << endl;
}
if (str1 == str3) {
cout << str1 << " is equal to " << str3 << endl;
} else if (str1 < str3) {
cout << str1 << " is smaller than " << str3 << endl;
} else {
cout << str1 << " is larger than " << str3 << endl;
}
return 0;
}
Output:
apple is smaller than banana
apple is equal to apple
2.3.2. Benefits of Using Comparison Operators
- Readability: Comparison operators provide a more readable and intuitive way to compare strings.
- String Class Integration: They are seamlessly integrated with the C++
string
class. - Conciseness: Comparison operators allow for concise and straightforward code.
3. Practical Examples of Lexicographical String Comparison
To illustrate the practical applications of lexicographical string comparison, let’s consider several examples:
3.1. Sorting a List of Names
Sorting a list of names alphabetically is a common task that requires lexicographical string comparison. Here’s an example:
#include <iostream>
#include <string>
#include <vector>
#include <algorithm>
using namespace std;
int main() {
vector<string> names = {"Charlie", "Alice", "Bob", "David"};
sort(names.begin(), names.end());
cout << "Sorted names:" << endl;
for (const string& name : names) {
cout << name << endl;
}
return 0;
}
Output:
Sorted names:
Alice
Bob
Charlie
David
In this example, the sort()
function from the <algorithm>
library is used to sort the vector of names. The sort()
function uses lexicographical comparison by default to arrange the strings in ascending order.
3.2. Implementing a Dictionary
Lexicographical order is fundamental to implementing a dictionary. Here’s a basic example:
#include <iostream>
#include <string>
#include <map>
using namespace std;
int main() {
map<string, string> dictionary;
dictionary["apple"] = "A round fruit with red, green, or yellow skin and crisp flesh.";
dictionary["banana"] = "A long, curved fruit with yellow skin and soft, sweet flesh.";
dictionary["orange"] = "A citrus fruit with a thick, orange skin and juicy pulp.";
cout << "Dictionary:" << endl;
for (const auto& pair : dictionary) {
cout << pair.first << ": " << pair.second << endl;
}
return 0;
}
Output:
Dictionary:
apple: A round fruit with red, green, or yellow skin and crisp flesh.
banana: A long, curved fruit with yellow skin and soft, sweet flesh.
orange: A citrus fruit with a thick, orange skin and juicy pulp.
In this example, the map
data structure automatically sorts the keys (words) in lexicographical order, making it easy to implement a dictionary.
3.3. Validating User Input
Lexicographical comparison can be used to validate user input, ensuring that it conforms to specific patterns or rules. For example, you can check if a username is valid by ensuring it starts with a letter and contains only alphanumeric characters:
#include <iostream>
#include <string>
using namespace std;
bool isValidUsername(const string& username) {
if (username.empty() || !isalpha(username[0])) {
return false;
}
for (char c : username) {
if (!isalnum(c)) {
return false;
}
}
return true;
}
int main() {
string username1 = "Alice123";
string username2 = "123Bob";
string username3 = "Charlie!";
cout << username1 << " is valid: " << isValidUsername(username1) << endl;
cout << username2 << " is valid: " << isValidUsername(username2) << endl;
cout << username3 << " is valid: " << isValidUsername(username3) << endl;
return 0;
}
Output:
Alice123 is valid: 1
123Bob is valid: 0
Charlie! is valid: 0
In this example, the isValidUsername()
function checks if the username starts with a letter and contains only alphanumeric characters. This ensures that the username is valid according to the specified rules.
4. Advanced Techniques and Considerations
While basic lexicographical string comparison is straightforward, there are several advanced techniques and considerations that can improve efficiency and handle specific use cases.
4.1. Case-Insensitive Comparison
By default, lexicographical comparison is case-sensitive, meaning that “apple” is different from “Apple”. To perform a case-insensitive comparison, you can convert both strings to lowercase (or uppercase) before comparing them:
#include <iostream>
#include <string>
#include <algorithm>
using namespace std;
string toLowercase(const string& str) {
string result = str;
transform(result.begin(), result.end(), result.begin(), ::tolower);
return result;
}
int main() {
string str1 = "Apple";
string str2 = "apple";
string lowerStr1 = toLowercase(str1);
string lowerStr2 = toLowercase(str2);
if (lowerStr1 == lowerStr2) {
cout << str1 << " is equal to " << str2 << " (case-insensitive)" << endl;
} else if (lowerStr1 < lowerStr2) {
cout << str1 << " is smaller than " << str2 << " (case-insensitive)" << endl;
} else {
cout << str1 << " is larger than " << str2 << " (case-insensitive)" << endl;
}
return 0;
}
Output:
Apple is equal to apple (case-insensitive)
In this example, the toLowercase()
function converts both strings to lowercase before comparing them, ensuring a case-insensitive comparison.
4.2. Locale-Aware Comparison
Lexicographical comparison is typically based on ASCII values, which may not be suitable for all languages. To perform a locale-aware comparison that considers language-specific sorting rules, you can use the <locale>
library:
#include <iostream>
#include <string>
#include <locale>
#include <algorithm>
using namespace std;
int main() {
locale loc("en_US.UTF-8"); // Example locale: US English with UTF-8 encoding
string str1 = "cote";
string str2 = "côte"; // "cote" with a circumflex
const collate<char>& coll = use_facet<collate<char>>(loc);
if (coll.compare(str1.data(), str1.data() + str1.length(),
str2.data(), str2.data() + str2.length()) < 0) {
cout << str1 << " is smaller than " << str2 << " (locale-aware)" << endl;
} else if (coll.compare(str1.data(), str1.data() + str1.length(),
str2.data(), str2.data() + str2.length()) > 0) {
cout << str1 << " is larger than " << str2 << " (locale-aware)" << endl;
} else {
cout << str1 << " is equal to " << str2 << " (locale-aware)" << endl;
}
return 0;
}
The <locale>
header provides tools for internationalization, allowing you to perform string comparisons that respect language-specific rules.
4.3. Performance Considerations
When comparing long strings or performing a large number of comparisons, performance becomes a critical factor. Here are some tips to optimize performance:
- Minimize String Copies: Avoid unnecessary string copies, as they can be expensive. Use references or pointers to pass strings to functions.
- Early Exit: If you only need to determine if one string is smaller or larger than another, exit the comparison as soon as you find a difference.
- Use
strncmp()
for Prefix Comparisons: If you only need to compare a specific number of characters, use thestrncmp()
function, which can be more efficient than comparing the entire string.
5. Comparing Strings Lexicographically in C++: A Summary Table
Feature | compare() Function |
strcmp() Function |
Comparison Operators |
---|---|---|---|
Data Type | C++ string objects |
C-style strings (null-terminated character arrays) | C++ string objects |
Library | <string> |
<cstring> |
N/A (built-in) |
Return Value | 0 if equal, negative if smaller, positive if larger | 0 if equal, negative if smaller, positive if larger | true or false (for ==, !=, >, <, >=, <=) |
Syntax | string1.compare(string2) |
strcmp(cstr1, cstr2) |
string1 == string2 , string1 < string2 , etc. |
Ease of Use | Easy | Requires C-style strings or conversion | Very Easy |
Readability | Good | Less readable | Excellent |
Case Sensitivity | Case-sensitive by default | Case-sensitive | Case-sensitive |
Locale Support | Requires additional locale settings | Limited locale support | Requires additional locale settings |
Performance | Generally good | Efficient for C-style strings | Generally good, may vary based on compiler optimization |
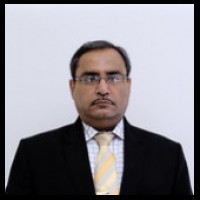
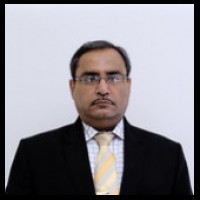
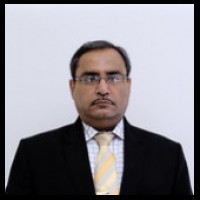
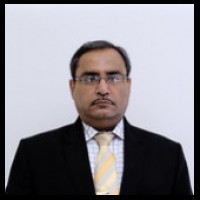
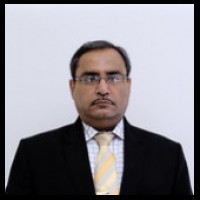
6. Common Mistakes and How to Avoid Them
When working with lexicographical string comparison in C++, it’s easy to make mistakes. Here are some common pitfalls and how to avoid them:
6.1. Incorrectly Using strcmp()
with C++ Strings
A common mistake is to use strcmp()
directly with C++ string
objects without converting them to C-strings first. This can lead to unexpected behavior or compilation errors.
How to Avoid: Always use the c_str()
method to convert C++ strings to C-strings before passing them to strcmp()
:
#include <iostream>
#include <cstring>
#include <string>
using namespace std;
int main() {
string str1 = "apple";
string str2 = "banana";
// Correct way to use strcmp() with C++ strings:
int result = strcmp(str1.c_str(), str2.c_str());
// Incorrect way:
// int result = strcmp(str1, str2); // This will cause an error
return 0;
}
6.2. Ignoring Case Sensitivity
Failing to account for case sensitivity can lead to incorrect comparisons, especially when dealing with user input or data from different sources.
How to Avoid: If case sensitivity is not required, convert both strings to lowercase or uppercase before comparing them.
6.3. Neglecting Locale-Specific Sorting Rules
Assuming that ASCII values are sufficient for all languages can lead to incorrect sorting and comparison results.
How to Avoid: Use the <locale>
library to perform locale-aware comparisons when dealing with multilingual data.
6.4. Overlooking Null Termination
When working with C-style strings, forgetting to null-terminate the character array can lead to buffer overflows or incorrect comparisons.
How to Avoid: Always ensure that C-style strings are properly null-terminated.
6.5. Inefficient String Copies
Unnecessary string copies can degrade performance, especially when dealing with long strings or a large number of comparisons.
How to Avoid: Use references or pointers to pass strings to functions and minimize string copies.
7. Choosing the Right Method for String Comparison
Selecting the appropriate method for string comparison depends on the specific requirements of your application. Here’s a guide to help you choose the right approach:
7.1. When to Use compare()
- C++ Strings: Use
compare()
when working with C++string
objects. - Comprehensive Comparison: When you need a comprehensive comparison that considers all characters in the strings.
- String Class Integration: When you want seamless integration with the C++
string
class.
7.2. When to Use strcmp()
- C-style Strings: Use
strcmp()
when working with C-style strings (null-terminated character arrays). - Performance: When performance is critical and you are already working with C-style strings.
- Legacy Code: When you need to maintain compatibility with legacy C code.
7.3. When to Use Comparison Operators
- Readability: Use comparison operators when you want the most readable and intuitive code.
- C++ Strings: When working with C++
string
objects. - Simplicity: When you need a simple and straightforward comparison.
Here’s a table summarizing the recommendations:
Scenario | Recommended Method(s) |
---|---|
C++ Strings | compare() , Comparison Operators |
C-style Strings | strcmp() |
Readability | Comparison Operators |
Performance (C-style Strings) | strcmp() |
Comprehensive Comparison | compare() |
8. FAQs About Lexicographical String Comparison in C++
Here are some frequently asked questions about lexicographical string comparison in C++:
Q1: What is lexicographical order?
A: Lexicographical order, also known as dictionary order, is a way of ordering strings based on the ASCII values of their characters. It’s the order in which strings would appear in a dictionary.
Q2: How is lexicographical comparison different from numerical comparison?
A: Lexicographical comparison compares strings character by character, while numerical comparison compares numbers based on their values. For example, “10” is lexicographically smaller than “2” because ‘1’ comes before ‘2’ in ASCII, but numerically, 10 is greater than 2.
Q3: Is lexicographical comparison case-sensitive?
A: Yes, by default, lexicographical comparison is case-sensitive. “apple” is different from “Apple”. To perform a case-insensitive comparison, convert both strings to lowercase or uppercase before comparing them.
Q4: How can I perform a case-insensitive lexicographical comparison in C++?
A: You can convert both strings to lowercase or uppercase before comparing them using functions like tolower()
or toupper()
from the <algorithm>
library.
Q5: What is the difference between compare()
and strcmp()
?
A: compare()
is a member function of the C++ string
class, while strcmp()
is a C-style string comparison function. compare()
works with C++ string
objects, while strcmp()
works with null-terminated character arrays (C-strings).
Q6: Can I use comparison operators (==, !=, >, <, >=, <=) to compare strings in C++?
A: Yes, C++ allows you to use comparison operators to compare strings lexicographically. These operators are overloaded for the string
class, providing a convenient and intuitive way to compare strings.
Q7: How can I compare strings in a locale-specific manner?
A: Use the <locale>
library to perform locale-aware comparisons that consider language-specific sorting rules.
Q8: What are some common mistakes to avoid when comparing strings in C++?
A: Common mistakes include incorrectly using strcmp()
with C++ strings, ignoring case sensitivity, neglecting locale-specific sorting rules, overlooking null termination, and inefficient string copies.
Q9: Which method should I use for string comparison in C++?
A: The choice of method depends on the specific requirements of your application. Use compare()
or comparison operators when working with C++ string
objects, and strcmp()
when working with C-style strings.
Q10: How can I improve the performance of string comparisons in C++?
A: Minimize string copies, use references or pointers to pass strings to functions, exit the comparison as soon as you find a difference, and use strncmp()
for prefix comparisons.
9. Conclusion
Lexicographical string comparison is a fundamental concept in C++ programming, essential for various applications such as sorting, searching, and data validation. This guide has explored different methods for performing string comparisons, including the compare()
function, the strcmp()
function, and comparison operators.
Understanding the nuances of each method, along with advanced techniques such as case-insensitive comparison and locale-aware comparison, allows developers to write efficient and accurate code. By avoiding common mistakes and choosing the right method for the task, you can ensure that your string comparisons are both effective and performant.
Whether you are sorting a list of names, implementing a dictionary, or validating user input, mastering lexicographical string comparison in C++ is a valuable skill for any programmer.
Ready to make smarter choices? Visit COMPARE.EDU.VN today to explore detailed comparisons and make informed decisions. Our comprehensive comparisons provide you with the insights you need to choose the best options for your specific needs. Don’t make decisions in the dark – let COMPARE.EDU.VN light the way!
For further assistance, contact us at:
Address: 333 Comparison Plaza, Choice City, CA 90210, United States
WhatsApp: +1 (626) 555-9090
Website: compare.edu.vn